Ra quyết định là khía cạnh quan trọng nhất của hầu hết các ngôn ngữ lập trình. Đúng như tên gọi, việc ra quyết định cho phép chúng ta chạy một khối mã cụ thể cho một quyết định cụ thể. Ở đây, các quyết định được đưa ra dựa trên tính hợp lệ của các điều kiện cụ thể.
Trong python, việc ra quyết định được thực hiện bằng các câu lệnh sau.
Câu lệnh | Mô tả |
---|---|
If | Câu lệnh if được sử dụng để kiểm tra một điều kiện cụ thể. Nếu điều kiện đúng, một khối mã (if-block) sẽ được thực thi. |
If – else | Câu lệnh if-else tương tự như câu lệnh if ngoại trừ thực tế là nó cũng cung cấp khối mã cho trường hợp sai của điều kiện cần kiểm tra. Nếu điều kiện được cung cấp trong câu lệnh if là sai thì câu lệnh else sẽ được thực thi. |
Câu lệnh If lồng nhau | Các câu lệnh if lồng nhau cho phép chúng ta sử dụng if ? câu lệnh else bên trong câu lệnh if bên ngoài. |
Thụt lề trong Python
Để dễ lập trình và đạt được sự đơn giản, python không cho phép sử dụng dấu ngoặc đơn cho khối mã. Trong Python, thụt lề được sử dụng để khai báo một khối. Nếu hai câu lệnh có cùng mức thụt lề thì chúng là một phần của cùng một khối.
Nói chung, bốn khoảng trắng được cung cấp để thụt lề các câu lệnh, đây là lượng thụt lề điển hình trong python.
Thụt lề là phần được sử dụng nhiều nhất trong ngôn ngữ python vì nó khai báo khối mã. Tất cả các câu lệnh của một khối đều có cùng mức thụt lề. Chúng ta sẽ xem quá trình thụt lề thực tế diễn ra như thế nào trong việc ra quyết định và những thứ khác trong python.
Câu lệnh if
Câu lệnh if được sử dụng để kiểm tra một điều kiện cụ thể và nếu điều kiện đó đúng, nó sẽ thực thi một khối mã được gọi là khối if. Điều kiện của câu lệnh if có thể là bất kỳ biểu thức logic hợp lệ nào có thể được đánh giá là đúng hoặc sai.
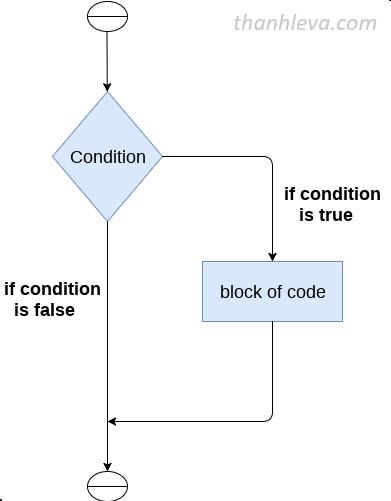
Cú pháp của câu lệnh if được đưa ra dưới đây.
if expression:
statement
Example 1
# Simple Python program to understand the if statement
num = int(input("enter the number:"))
# Here, we are taking an integer num and taking input dynamically
if num%2 == 0:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("The Given number is an even number")
Output:
enter the number: 10
The Given number is an even number
Example 2 : Chương trình in số lớn nhất trong 3 số
# Simple Python Program to print the largest of the three numbers.
a = int (input("Enter a: "));
b = int (input("Enter b: "));
c = int (input("Enter c: "));
if a>b and a>c:
# Here, we are checking the condition. If the condition is true, we will enter the block
print ("From the above three numbers given a is largest");
if b>a and b>c:
# Here, we are checking the condition. If the condition is true, we will enter the block
print ("From the above three numbers given b is largest");
if c>a and c>b:
# Here, we are checking the condition. If the condition is true, we will enter the block
print ("From the above three numbers given c is largest");
Output:
Enter a: 100
Enter b: 120
Enter c: 130
From the above three numbers given c is largest
Câu lệnh if-else
Câu lệnh if-else cung cấp một khối else kết hợp với câu lệnh if được thực thi trong trường hợp điều kiện sai.
Nếu điều kiện đúng thì khối if được thực thi. Ngược lại, khối else sẽ được thực thi.
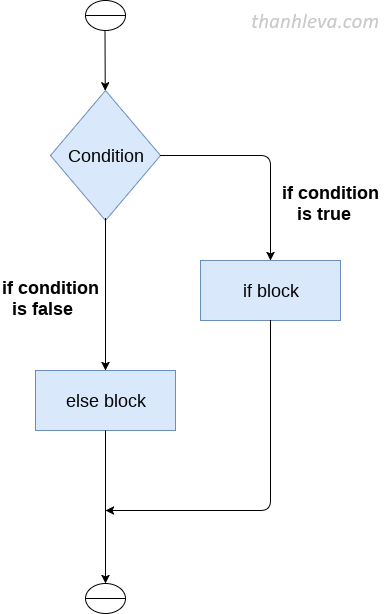
Cú pháp của câu lệnh if-else được đưa ra dưới đây.
if condition:
#block of statements
else:
#another block of statements (else-block)
Example 1: Chương trình kiểm tra một người có đủ điều kiện bầu cử hay không.
# Simple Python Program to check whether a person is eligible to vote or not.
age = int (input("Enter your age: "))
# Here, we are taking an integer num and taking input dynamically
if age>=18:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("You are eligible to vote !!");
else:
print("Sorry! you have to wait !!");
Output:
Enter your age: 90
You are eligible to vote !!
Example 2: Chương trình kiểm tra một số có phải là số chẵn hay không.
# Simple Python Program to check whether a number is even or not.
num = int(input("enter the number:"))
# Here, we are taking an integer num and taking input dynamically
if num%2 == 0:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("The Given number is an even number")
else:
print("The Given Number is an odd number")
Output:
enter the number: 10
The Given number is even number
Câu lệnh elif
Câu lệnh elif cho phép chúng ta kiểm tra nhiều điều kiện và thực thi khối câu lệnh cụ thể tùy thuộc vào điều kiện thực sự trong số đó. Chúng ta có thể có bất kỳ số lượng câu lệnh elif nào trong chương trình tùy theo nhu cầu của chúng ta. Tuy nhiên, sử dụng Elif là tùy chọn.
Câu lệnh elif hoạt động giống như câu lệnh bậc thang if-else-if trong C. Nó phải được nối tiếp bởi câu lệnh if.
Cú pháp của câu lệnh Elif được đưa ra dưới đây.
if expression 1:
# block of statements
elif expression 2:
# block of statements
elif expression 3:
# block of statements
else:
# block of statements
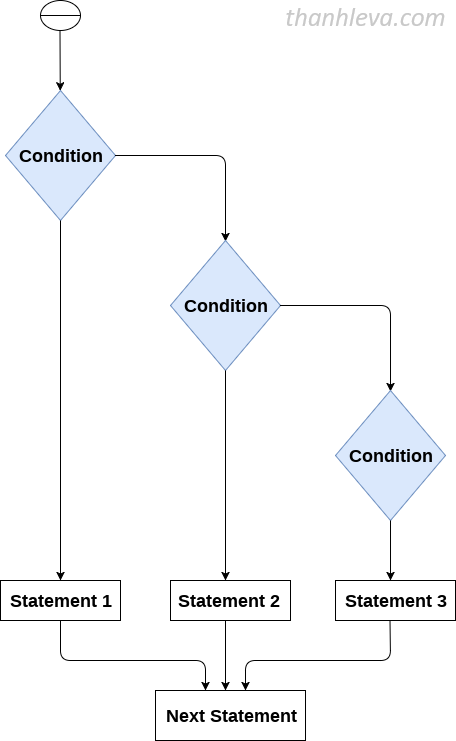
Example 1
# Simple Python program to understand elif statement
number = int(input("Enter the number?"))
# Here, we are taking an integer number and taking input dynamically
if number==10:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("The given number is equals to 10")
elif number==50:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("The given number is equal to 50");
elif number==100:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("The given number is equal to 100");
else:
print("The given number is not equal to 10, 50 or 100");
Output:
Enter the number?15
The given number is not equal to 10, 50 or 100
Example 2
# Simple Python program to understand elif statement
marks = int(input("Enter the marks? "))
# Here, we are taking an integer marks and taking input dynamically
if marks > 85 and marks <= 100:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("Congrats ! you scored grade A ...")
elif marks > 60 and marks <= 85:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("You scored grade B + ...")
elif marks > 40 and marks <= 60:
# Here, we are checking the condition. If the condition is true, we will enter the block
print("You scored grade B ...")
elif (marks > 30 and marks <= 40):
# Here, we are checking the condition. If the condition is true, we will enter the block
print("You scored grade C ...")
else:
print("Sorry you are fail ?")
Output:
Enter the marks? 89
Congrats ! you scored grade A ...